7 min to read
Buff Up Your Go Skills
Mastering Buffers for Peak Performance
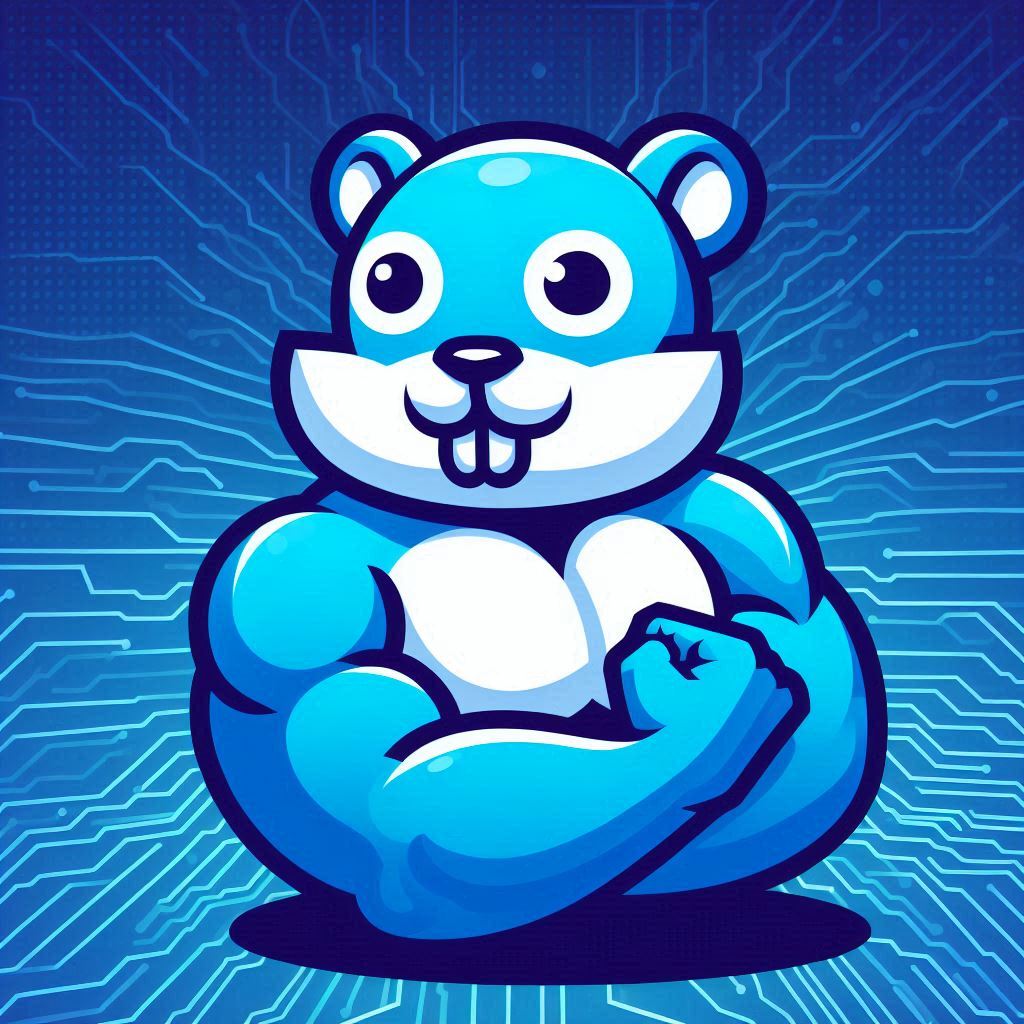
Let’s dive into one of those nifty little tools in the Go programming language toolbox: buffers. You might have heard of them, but what exactly are they, and why should you care?
What’s a Buffer Anyway?
So, picture this: you’ve got this chunk of data you need to handle in your Go program. It could be bytes, strings, or anything really. Now, instead of dealing with it directly, you toss it into this magic container called a buffer. Think of it like a storage unit for your data, where you can stash stuff away for safekeeping or manipulation.
Real-World Examples
Here are a few instances where buffers can come to the rescue:
- Network Communication: Ever sent or received data over a network? Buffers can help streamline that process, making it faster and more efficient.
- File I/O: Reading or writing files? Buffers can minimize those pesky disk I/O operations, saving you time and headaches.
- String Concatenation: Need to build a big string from smaller pieces? Buffers got your back, making the process smoother and less resource-intensive.
- Data Processing Pipelines: Buffers are like the glue that holds data processing pipelines together, allowing different parts of your program to work together seamlessly.
- HTTP Request/Response Handling: Handling HTTP requests and responses? Buffers can simplify the process, especially when dealing with large payloads.
Let’s Get Coding!
Alright, enough chit-chat. Let’s see some code in action! Below is a detailed example of using a buffer in Go for handling HTTP requests and responses:
package main
import (
"bytes"
"encoding/json"
"fmt"
"net/http"
)
type RequestData struct {
Name string `json:"name"`
Age int `json:"age"`
}
type ResponseData struct {
Message string `json:"message"`
}
func handleRequest(w http.ResponseWriter, r *http.Request) {
// Step 1: Create a Buffer
var buf bytes.Buffer
// Step 2: Read request body into the buffer
_, err := buf.ReadFrom(r.Body)
if err != nil {
http.Error(w, "Error reading request body", http.StatusInternalServerError)
return
}
// Step 3: Decode JSON data from the buffer
var requestData RequestData
err = json.Unmarshal(buf.Bytes(), &requestData)
if err != nil {
http.Error(w, "Error decoding JSON data", http.StatusBadRequest)
return
}
// Step 4: Process the request data
message := fmt.Sprintf("Hello, %s! You are %d years old.", requestData.Name, requestData.Age)
// Step 5: Create response data
responseData := ResponseData{
Message: message,
}
// Step 6: Encode response data as JSON
responseJSON, err := json.Marshal(responseData)
if err != nil {
http.Error(w, "Error encoding response data", http.StatusInternalServerError)
return
}
// Step 7: Write response
w.Header().Set("Content-Type", "application/json")
_, err = w.Write(responseJSON)
if err != nil {
http.Error(w, "Error writing response", http.StatusInternalServerError)
return
}
}
func main() {
http.HandleFunc("/", handleRequest)
fmt.Println("Server listening on port 8080...")
http.ListenAndServe(":8080", nil)
}
Breaking Down the Example
Let’s break down what’s happening in this code step-by-step:
-
Create a Buffer: We start by creating an instance of
bytes.Buffer
. This buffer will temporarily hold the data from the HTTP request body.var buf bytes.Buffer
-
Read Request Body into the Buffer: We read the entire request body into the buffer using
buf.ReadFrom(r.Body)
. This method reads from the request body until EOF and writes it to the buffer._, err := buf.ReadFrom(r.Body) if err != nil { http.Error(w, "Error reading request body", http.StatusInternalServerError) return }
-
Decode JSON Data from the Buffer: Once the data is in the buffer, we can easily decode it into our
RequestData
struct. We do this by usingjson.Unmarshal(buf.Bytes(), &requestData)
, which converts the JSON data into a Go struct.var requestData RequestData err = json.Unmarshal(buf.Bytes(), &requestData) if err != nil { http.Error(w, "Error decoding JSON data", http.StatusBadRequest) return }
-
Process the Request Data: Now that we have the request data in a structured format, we can process it. Here, we’re simply creating a greeting message.
message := fmt.Sprintf("Hello, %s! You are %d years old.", requestData.Name, requestData.Age)
-
Create Response Data: We prepare the response data by populating a
ResponseData
struct.responseData := ResponseData{ Message: message, }
-
Encode Response Data as JSON: Next, we convert the response data into JSON format using
json.Marshal(responseData)
. This prepares it for transmission back to the client.responseJSON, err := json.Marshal(responseData) if err != nil { http.Error(w, "Error encoding response data", http.StatusInternalServerError) return }
-
Write Response: Finally, we write the JSON response back to the client, setting the appropriate content type.
w.Header().Set("Content-Type", "application/json") _, err = w.Write(responseJSON) if err != nil { http.Error(w, "Error writing response", http.StatusInternalServerError) return }
Wrapping Up
Buffers might seem like a small detail, but they can make a big difference in the performance and readability of your Go code. So next time you’re faced with a data-handling dilemma, remember to reach for that trusty buffer and let it work its magic!
Comments